数据类型
基本类型
undefine,null,boolean,string,number
包装类型
boolean ,string,number都有相应的包装的类型Boolean,String,Number
对象类型
object,function,Array,Date,window…
类型检查
typeof
1
2
3typeof('123') "string"
typeof(new String()) "object"
typeof(null) "object"判断对象的类型:基本类型返回基本类型;对象返回 object
instanceof
基于原型链判断类型
1
2
3
4
5
6
7
8function Person(){};
function Student(){};
Student.prototype = new Person();
var stu = new Student();
var per = new Person();
stu instanceof Student true
per instanceof Person true
stu instanceof Person true
类型转换
+,-
1
232+'32' '3232'
32-'1' 31通过这种机制来进行string,number相互转换
==
1
2
3
4'1.23'==1.23 true
0 == false
null == undefine
new Object() == new Object() false转换成目标类型,再比较
===
1
2null === null true
undefined === undefined true类型相同并且值相等
表达式
数组,对象初始化表达式
1
2
3[1,2] //数组表达式 new Array(1,2)
[1,,,4] [1,undefined,undefined,4]
{x:1,y:2} //对象表达式 var o = new Object();o.x=1;o.y=2;函数表达式
1
2var fe = function(){};
(function(){console.log('hello world!');})();属性访问表达式
1
2
3var a = {x:100,y:50};
a.x //访问对象的属性表达式
a['x']调用表达式
1
func()
对象创建表达式
1
new Func(1,2);
this
在不同环境下,同一个函数在不同的调用方式下,this不同
作为对象的方法上的this
1
2
3
4
5
6
7var o = {
prop:37,
f:function(){
return this.prop;
}
};
console.log(o.f()); //this指向 函数 o对象原型链上的this
1
2
3
4
5var o = { f:function(){return this.a+this.b}};
var p = Object.create(o);
p.a = 1
p.b =4;
console(p.f()); //5 this 执行函数P通过new 的方式调用
1
2
3
4
5
6function A(){
this.a=37;
return {a:38};
}
o= new A();
console.log(o.a);call,apply
修改this的指向
1
2
3
4
5function add(c,d){
return this.a+this.b+c+d;
}
var o={a:1,b:2};
add.call(o,5,7);bind
一次绑定多次使用
1
2
3
4
5
6
7function f(){
return this.a;
}
var g = f.bind({a:"test"});
console.log(g());//test
var o = {a:37,f:f,g:g};
console.log(o.f(),o.g()); //37,test
闭包
私有全局变量
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34function outer(){
var local = 30;
return local;
}
outer();//30 方法调用完 local局部变量被回收了。
function outer(){
var local = 30 ;
return function(){
return local;
}
}
var func = outer();
func();//30 在outer调用完,local没有被回收。
(function(){
var userId ='123';
var password = '6789@jkl';
var Artery = {};
function getUserName(){
return userId;
}
function getPassword(){
return password
}
Artery.getUserId(){
return getUserName();
}
Artery.getPassword(){
return getPassword();
}
window.Artery = Artery;
}());
Artery.getUserId();OOP
继承,封装,多态,抽象
原型链
1
2
3
4
5
6
7
8
9function foo(){};每个函数都有一个prototype属性;
foo.prototype.x = 1;
foo.prototype //prototype的属性
{
constructor: foo,
_proto_:Object.prototype,
x:1
}
var obj = new foo();
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
function Person(name,age){
this.name = name;
this.age = age;
}
Person.protype.hi = function(){
console.log('hi,my name is '+ this.name+'age:'+this.age);
}
Person.protype.less_num = 2
Person.protype.
function Student(name,age,className){
Person.call(this,name,age)
this.className =className;
}
Student.protype = Object.create(Person.protype);
Student.protype.constructor = Student;
Student.protype.hi = function(){
console.log('hi my name is '+ this.name+'I am '+age+ 'and from '+ this.className);
}
var bosn = new Student("bosn",20,1);
bosn.hi();
bosn.walk();
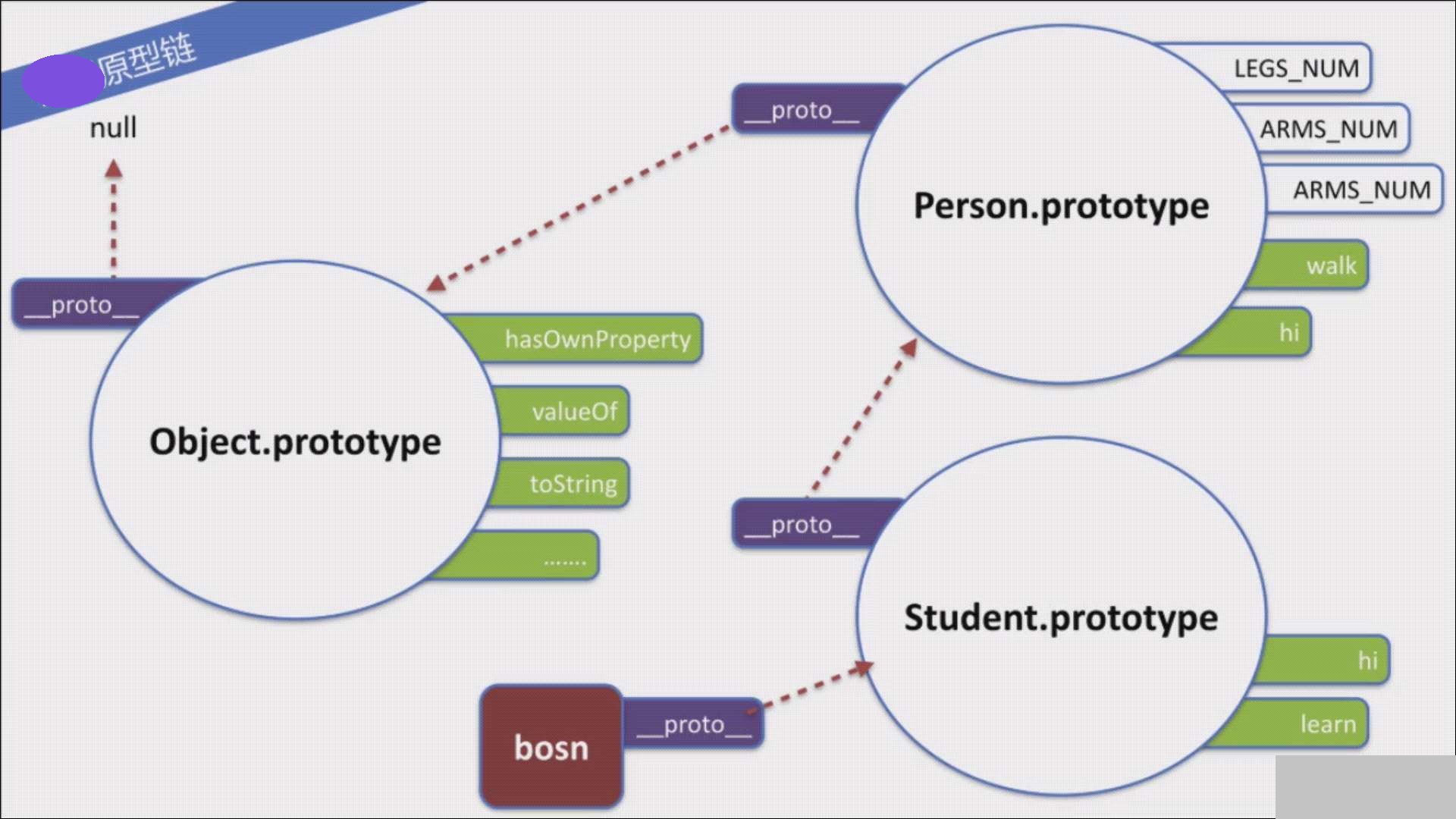
正则表达式
具体语法参考正则表达式文章。